NPM모듈은 모듈로 import해서 함수나 클래스를 사용하는것 이외에도 일반적인 실행 파일로도 사용이 가능 합니다.
NPM을 모듈 bin mode
- npm은 보통 library 형태로 많이 사용하지만, 일반적인 실행 파일과 동일한 방식으로도 사용이 가능하다.
- 대표적인 예로는 create react app과 같은 모듈을 볼 수 있다.
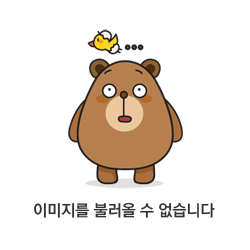
- 위 create react app 모둘의 실행 방식이다. javascript 파일이나, typescript 파일에서 import 하는 방식이 아닌, 직접 실행하는 듯한 모습을 볼 수 있다.
- npx는 모듈이 설치되어 있는 경우 실행을 아닌 경우 바로 설치해 주는 기능을 하며 실행 후 실행을 위해서 설치한 파일을 남기지 않고 삭제됩니다. 필요한 기능만 실행 후 깔끔하게 파일은 정리해 버리게 됩니다.
- npx를 많이 사용하는 이유는 package 설치를 알아서 해주는 부분과, 모든 굳이 global로 일회성으로 사영되는 package 등을 global로 설치하지 않아도 되기 때문이다.
NPM bin module의 내부
- npm bin module도 실행 파일처럼 사용하지만 일반적인 npm과 그 모양은 비슷하다. npm에 따라 약간 실행 방식은 다르긴 하지만, bin module의 실행도 결과 node.js에 bin module 내부의. js 코드를 실행시키는 방식입니다.
- 일반 create react app의 package.json을 살펴보겠습니다.
{
"name": "create-react-app",
"version": "5.0.1",
"keywords": [
"react"
],
"description": "Create React apps with no build configuration.",
"repository": {
"type": "git",
"url": "<https://github.com/facebook/create-react-app.git>",
"directory": "packages/create-react-app"
},
"license": "MIT",
"engines": {
"node": ">=14"
},
"bugs": {
"url": "<https://github.com/facebook/create-react-app/issues>"
},
"files": [
"index.js",
"createReactApp.js"
],
"bin": {
"create-react-app": "./index.js"
},
"scripts": {
"test": "cross-env FORCE_COLOR=true jest"
},
- 여기서 특이한 부분을 볼 수 있는데, bin이라는 항목을 볼 수 있습니다.
- bin이 실행시킬 파일에 대한 정보를 기술합니다.
- "create-react-app": "./index.js"
- create-react-app 이 실행 command이며 실제로 실행시킬 파일이 “./index.js”입니다.
create-react-app myapp
- 위와 같은 실행 한다면 실제로 내부에서는
nodejs ./index.js myapp
- 위와 같이 실행됩니다.
- 하지만, 문제가 있습니다. create-react-app이라는 프로그램이 실제로 시스템에 설치되어 있지 않으면 create-react-app 이라는 이름의 command는 없는 것으로 나타나게 됩니다.
- user@computer create-react-app zsh: command not found: create-react-app
- node.js를 설치하게 되는 경우, node_modules/bin이라는 폴더가 system의 path에 설정됩니다.
- npm install -g create-react-app으로 global로 설치하게 되면
- bin 폴더 내부에 create-react-app이라는 파일이 하나 생성되어 있음을 볼 수 있습니다. osx 기준으로 이 파일은 sh파일로 shell command를 실행시킬 수 있는 파일입니다.
!/usr/bin/env node
/**
* Copyright (c) 2015-present, Facebook, Inc.
*
* This source code is licensed under the MIT license found in the
* LICENSE file in the root directory of this source tree.
*/
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// /!\\ DO NOT MODIFY THIS FILE /!\\
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
//
// create-react-app is installed globally on people's computers. This means
// that it is extremely difficult to have them upgrade the version and
// because there's only one global version installed, it is very prone to
// breaking changes.
//
// The only job of create-react-app is to init the repository and then
// forward all the commands to the local version of create-react-app.
//
// If you need to add a new command, please add it to the scripts/ folder.
//
// The only reason to modify this file is to add more warnings and
// troubleshooting information for the `create-react-app` command.
//
// Do not make breaking changes! We absolutely don't want to have to
// tell people to update their global version of create-react-app.
//
// Also be careful with new language features.
// This file must work on Node 0.10+.
//
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// /!\\ DO NOT MODIFY THIS FILE /!\\
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
'use strict';
const currentNodeVersion = process.versions.node;
const semver = currentNodeVersion.split('.');
const major = semver[0];
if (major < 14) {
console.error(
'You are running Node ' +
currentNodeVersion +
'.\\n' +
'Create React App requires Node 14 or higher. \\n' +
'Please update your version of Node.'
);
process.exit(1);
}
const { init } = require('./createReactApp');
init();
- create-react-app command를 terminal에서 실해 시키면 아래와 같은 단계가 진행됩니다.
- !/usr/bin/env node → /usr/bin/env 에 있는 node 이 파일을 실행시켜라입니다.
- const { init } = require('./createReactApp'); →./createReactApp을 import 합니다.
- createReactApp의 init 함수를 호출합니다.
'node.js' 카테고리의 다른 글
NPM 모듈 개발 (0) | 2023.10.11 |
---|---|
NPM 개발 준비 (0) | 2023.09.24 |
NPM 이란 (0) | 2023.09.23 |
NPM을 만들어 보자 (0) | 2023.09.23 |